(AI translated)
Yesterday, I wanted to open an old file and pfff…. A quick analysis showed that the disk is dying, although SMART says everything is fine. Shit. But it’s a good opportunity to talk about how my disk farm is organized. I have already written that my home computer’s disks are gradually migrating to smaller ones. The disk that just arrived was large (in every sense), but now it will migrate to smaller ones.
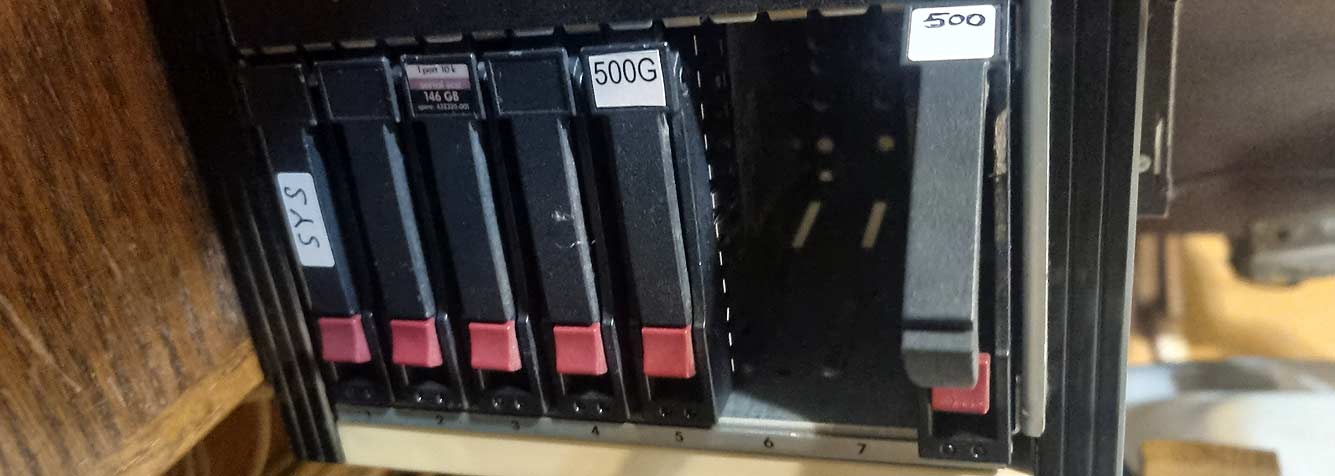
Mechanically, it’s a basket from some server. It was designed for SAS disks but is mechanically and electrically compatible with regular SATA disks. I just had to re-solder the cable to connect it not to the SAS RAID controller but to a regular SATA controller. Disk #1 is the system disk, a Samsung SSD. Disks #2, #3, and #4 are Disk X, a simple stripe RAID for temporary files (where standard TMP/TEMP and various programs’ SCRATCH files fall). Disk #5 with the 500 sticker is the new ‘My Documents’ disk. Disk #8 is not inserted.
The whole chaos looks like this:
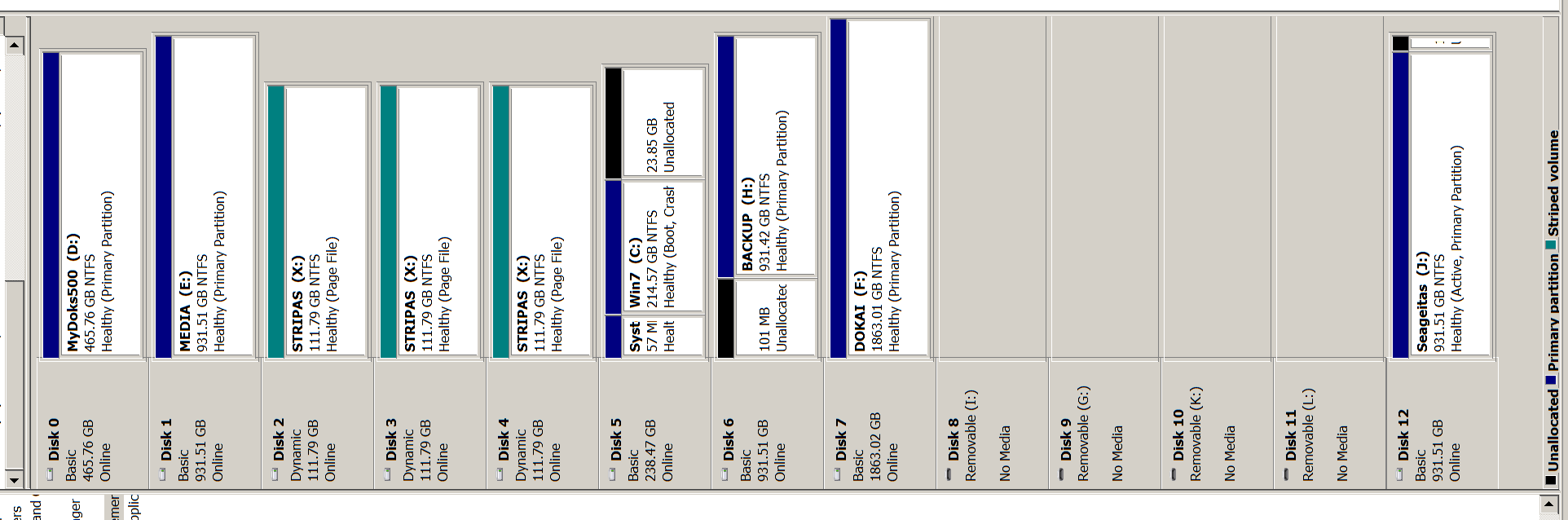
There are also the old ‘My Docs,’ ‘Media,’ and ‘Backup’ disks. They are currently of large format. The ‘Steam’ games disk was removed because document copying was being done at the time of the picture. And disk ‘J’ is a backup disk connected via USB – I was looking for a copy of the corrupted file. The ‘Backup’ disk is intended for Windows’ own backup. However, it is almost useless. I constantly find that the backup did not happen or something similar.
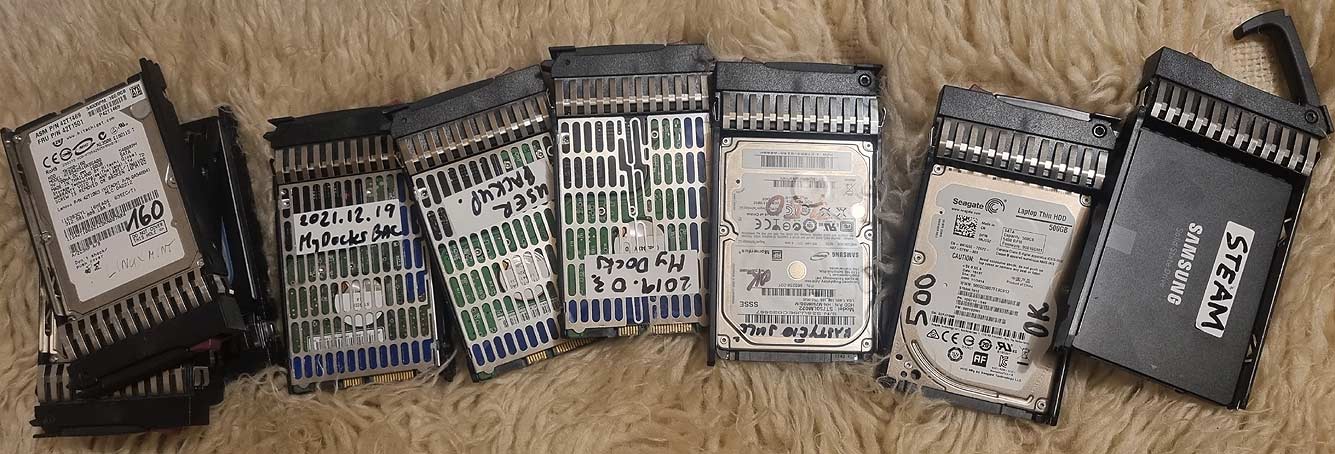
Here’s how the floppy collection looks. I liked the basket’s mechanics, so I collected handles. And where do the disks come from? Well, when you change the working laptop disks to SSDs, you format the disks and use them. And they got collected from somewhere. That’s why they are so small – old and ‘working’.